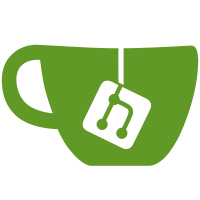
Create a MessageUtil class to handle sending and receiving messages between the extension and the chat panel. Add a function to send messages to the extension and register message handlers to receive messages from the extension. When the user clicks the send button, send the message to the extension. When the extension sends a message, add it to the chat UI as a bot message.
46 lines
1.3 KiB
TypeScript
46 lines
1.3 KiB
TypeScript
|
|
// @ts-ignore
|
|
const vscodeApi = window.acquireVsCodeApi();
|
|
|
|
class MessageUtil {
|
|
handlers: { [x: string]: any; };
|
|
constructor() {
|
|
this.handlers = {};
|
|
}
|
|
|
|
// Register a message handler for a specific message type
|
|
registerHandler(messageType: string, handler: { (message: { text: string; }): void; (message: { text: string; }): void; }) {
|
|
if (!this.handlers[messageType]) {
|
|
this.handlers[messageType] = [];
|
|
}
|
|
this.handlers[messageType].push(handler);
|
|
}
|
|
|
|
// Unregister a message handler for a specific message type
|
|
unregisterHandler(messageType: string | number, handler: any) {
|
|
if (this.handlers[messageType]) {
|
|
this.handlers[messageType] = this.handlers[messageType].filter(
|
|
(h: any) => h !== handler
|
|
);
|
|
}
|
|
}
|
|
|
|
// Handle a received message
|
|
handleMessage(message: { command: string | number; }) {
|
|
console.log("handleMessage", message);
|
|
const handlers = this.handlers[message.command];
|
|
if (handlers) {
|
|
handlers.forEach((handler: (arg0: { command: string | number; }) => any) => handler(message));
|
|
}
|
|
}
|
|
|
|
// Send a message to the VSCode API
|
|
sendMessage(message: { command: string; text: string; }) {
|
|
console.log("sendMessage", message);
|
|
vscodeApi.postMessage(message);
|
|
}
|
|
}
|
|
|
|
// Export the MessageUtil class as a module
|
|
export default MessageUtil;
|