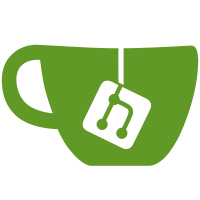
## Review A dedicated reviewer checked the rule description successfully for: - [ ] logical errors and incorrect information - [ ] information gaps and missing content - [ ] text style and tone - [ ] PR summary and labels follow [the guidelines](https://github.com/SonarSource/rspec/#to-modify-an-existing-rule) --------- Co-authored-by: Loris S <91723853+loris-s-sonarsource@users.noreply.github.com> Co-authored-by: leonardo-pilastri-sonarsource <115481625+leonardo-pilastri-sonarsource@users.noreply.github.com>
86 lines
1.8 KiB
Plaintext
86 lines
1.8 KiB
Plaintext
Ensuring the variable `myObject` has a value resolves the issue:
|
|
|
|
[source,java,diff-id=1,diff-type=compliant]
|
|
----
|
|
public void method() {
|
|
Object myObject = new Object();
|
|
System.out.println(myObject.toString()); // Compliant: myObject is not null
|
|
}
|
|
----
|
|
|
|
Preventing the non-compliant code to be executed by returning early:
|
|
|
|
[source,java,diff-id=2,diff-type=compliant]
|
|
----
|
|
public void method(Object input)
|
|
{
|
|
if (input == null)
|
|
{
|
|
return;
|
|
}
|
|
System.out.println(input.toString()); // Compliant: if 'input' is null, this is unreachable
|
|
}
|
|
----
|
|
|
|
Ensuring that no unboxing of `null` value can happen resolves the issue
|
|
|
|
[source,java,diff-id=3,diff-type=compliant]
|
|
----
|
|
public boolean method() {
|
|
Boolean boxed = true;
|
|
return boxed; // Compliant
|
|
}
|
|
----
|
|
|
|
Ensuring that both `conn` and `stmt` are not `null` resolves the issue:
|
|
|
|
[source,java,diff-id=4,diff-type=compliant]
|
|
----
|
|
Connection conn = null;
|
|
Statement stmt = null;
|
|
try {
|
|
conn = DriverManager.getConnection(DB_URL,USER,PASS);
|
|
stmt = conn.createStatement();
|
|
// ...
|
|
} catch(Exception e) {
|
|
e.printStackTrace();
|
|
} finally {
|
|
if (stmt != null) {
|
|
stmt.close(); // Compliant
|
|
}
|
|
if (conn != null) {
|
|
conn.close(); // Compliant
|
|
}
|
|
}
|
|
----
|
|
|
|
Checking the returned value of `getName()` resolves the issue:
|
|
|
|
[source,java,diff-id=5,diff-type=compliant]
|
|
----
|
|
@CheckForNull
|
|
String getName() {...}
|
|
|
|
public boolean isNameEmpty() {
|
|
String name = getName();
|
|
if (name != null) {
|
|
return name.length() == 0; // Compliant
|
|
} else {
|
|
// ...
|
|
}
|
|
}
|
|
----
|
|
|
|
Ensuring that the provided `color` is not `null` resolves the issue:
|
|
|
|
[source,java,diff-id=6,diff-type=compliant]
|
|
----
|
|
private void merge(@Nonnull Color firstColor, @Nonnull Color secondColor) {...}
|
|
|
|
public void append(@CheckForNull Color color) {
|
|
if (color != null) {
|
|
merge(currentColor, color); // Compliant
|
|
}
|
|
}
|
|
----
|