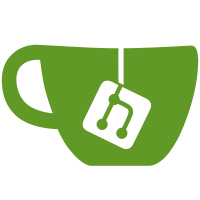
Inline adoc files when they are included exactly once. Also fix language tags because this inlining gives us better information on what language the code is written in.
57 lines
1.4 KiB
Plaintext
57 lines
1.4 KiB
Plaintext
== Why is this an issue?
|
|
|
|
include::../description.adoc[]
|
|
|
|
|
|
=== Noncompliant code example
|
|
|
|
[source,kotlin]
|
|
----
|
|
val p = Pattern.compile("^$", Pattern.MULTILINE) // Noncompliant
|
|
val r = Regex("^$", RegexOption.MULTILINE) // Noncompliant
|
|
|
|
// Alternatively
|
|
val p = Pattern.compile("(?m)^$") // Noncompliant
|
|
val r = Regex("(?m)^$") // Noncompliant
|
|
|
|
fun containsEmptyLines(str: String) : Boolean {
|
|
return p.matcher(str).find()
|
|
}
|
|
|
|
fun containsEmptyLinesKotlin(str: String) = r.find(str) != null
|
|
|
|
// ...
|
|
println(containsEmptyLines("a\n\nb")) // correctly prints 'true'
|
|
println(containsEmptyLinesKotlin("a\n\nb")) // correctly prints 'true'
|
|
|
|
println(containsEmptyLines("")) // incorrectly prints 'false'
|
|
println(containsEmptyLinesKotlin("")) // incorrectly prints 'false'
|
|
----
|
|
|
|
|
|
|
|
=== Compliant solution
|
|
|
|
[source,kotlin]
|
|
----
|
|
val p = Pattern.compile("^$", Pattern.MULTILINE) // Noncompliant
|
|
val r = Regex("^$", RegexOption.MULTILINE) // Noncompliant
|
|
|
|
fun containsEmptyLines(str: String) : Boolean {
|
|
return p.matcher(str).find() || str.isEmpty()
|
|
}
|
|
|
|
fun containsEmptyLinesKotlin(str: String) = r.find(str) != null || str.isEmpty()
|
|
|
|
// ...
|
|
println(containsEmptyLines("a\n\nb")) // correctly prints 'true'
|
|
println(containsEmptyLinesKotlin("a\n\nb")) // correctly prints 'true'
|
|
|
|
println(containsEmptyLines("")) // correctly prints 'true'
|
|
println(containsEmptyLinesKotlin("")) // correctly prints 'true'
|
|
----
|
|
|
|
|
|
|
|
include::../implementation.adoc[]
|