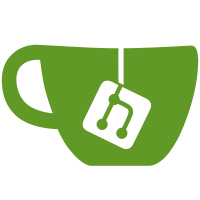
Inline adoc files when they are included exactly once. Also fix language tags because this inlining gives us better information on what language the code is written in.
72 lines
1.8 KiB
Plaintext
72 lines
1.8 KiB
Plaintext
== Why is this an issue?
|
|
|
|
In records, the default behavior of the ``++equals()++`` method is to check the equality by field values. This works well for primitive fields or fields, whose type overrides ``++equals()++``, but this behavior doesn't work as expected for array fields.
|
|
|
|
|
|
By default, array fields are compared by their reference, and overriding ``++equals()++`` is highly appreciated to achieve the deep equality check. The same strategy applies to ``++hashcode()++`` and ``++toString()++`` methods.
|
|
|
|
|
|
This rule reports an issue if a record class has an array field and is not overriding ``++equals()++``, ``++hashcode()++`` or ``++toString()++`` methods.
|
|
|
|
|
|
=== Noncompliant code example
|
|
|
|
[source,java]
|
|
----
|
|
record Person(String[] names, int age) {} // Noncompliant
|
|
----
|
|
|
|
|
|
=== Compliant solution
|
|
|
|
[source,java]
|
|
----
|
|
record Person(String[] names, int age) {
|
|
@Override
|
|
public boolean equals(Object o) {
|
|
if (this == o) return true;
|
|
if (o == null || getClass() != o.getClass()) return false;
|
|
Person person = (Person) o;
|
|
return age == person.age && Arrays.equals(names, person.names);
|
|
}
|
|
|
|
@Override
|
|
public int hashCode() {
|
|
int result = Objects.hash(age);
|
|
result = 31 * result + Arrays.hashCode(names);
|
|
return result;
|
|
}
|
|
|
|
@Override
|
|
public String toString() {
|
|
return "Person{" +
|
|
"names=" + Arrays.toString(names) +
|
|
", age=" + age +
|
|
'}';
|
|
}
|
|
}
|
|
----
|
|
|
|
|
|
== Resources
|
|
|
|
* https://docs.oracle.com/javase/specs/jls/se16/html/jls-8.html#jls-8.10[Records specification]
|
|
|
|
ifdef::env-github,rspecator-view[]
|
|
|
|
'''
|
|
== Implementation Specification
|
|
(visible only on this page)
|
|
|
|
=== Message
|
|
|
|
Override [equals|hashcode|toString] to consider array's content in the method
|
|
|
|
|
|
=== Highlighting
|
|
|
|
record declaration
|
|
|
|
|
|
endif::env-github,rspecator-view[]
|