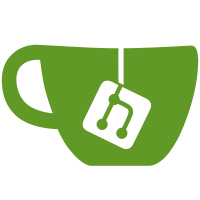
When an include is not surrounded by empty lines, its content is inlined on the same line as the adjacent content. That can lead to broken tags and other display issues. This PR fixes all such includes and introduces a validation step that forbids introducing the same problem again.
88 lines
1.8 KiB
Plaintext
88 lines
1.8 KiB
Plaintext
== Why is this an issue?
|
|
|
|
Shadowing parent class members by creating properties and methods with the same signatures as non-``++virtual++`` parent class members can result in seemingly strange behavior if an instance of the child class is cast to the parent class. In such cases, the parent class' code will be executed instead of the code in the child class, confusing callers and potentially causing hard-to-find bugs.
|
|
|
|
|
|
Instead the child class member should be renamed.
|
|
|
|
|
|
=== Noncompliant code example
|
|
|
|
[source,csharp]
|
|
----
|
|
public class Fruit
|
|
{
|
|
public double GetCost()
|
|
{
|
|
return 3.5;
|
|
}
|
|
}
|
|
|
|
public class Raspberry : Fruit
|
|
{
|
|
public new double GetCost() // Noncompliant
|
|
{
|
|
return 7.5;
|
|
}
|
|
}
|
|
|
|
// ...
|
|
var r = new Raspberry();
|
|
var f = (Fruit) r;
|
|
Console.WriteLine(r.GetCost()); // prints 7.5
|
|
Console.WriteLine(f.GetCost()); // prints 3.5; there's only one instance but different code executes depending on cast
|
|
----
|
|
|
|
|
|
=== Compliant solution
|
|
|
|
[source,csharp]
|
|
----
|
|
public class Fruit
|
|
{
|
|
public double GetCost()
|
|
{
|
|
return 3.5;
|
|
}
|
|
}
|
|
|
|
public class Raspberry : Fruit
|
|
{
|
|
public double GetInflatedCost()
|
|
{
|
|
return 7.5;
|
|
}
|
|
}
|
|
|
|
// ...
|
|
var r = new Raspberry();
|
|
var f = (Fruit) r;
|
|
Console.WriteLine(r.GetCost()); // prints 3.5
|
|
Console.WriteLine(f.GetCost()); // prints 3.5; same code executes every time
|
|
Console.WriteLine(r.GetInflatedCost()); // prints 7.5
|
|
----
|
|
|
|
|
|
=== Exceptions
|
|
|
|
This rule ignores ``++private++`` parent class members, and any method named ``++Equals++``.
|
|
|
|
|
|
ifdef::env-github,rspecator-view[]
|
|
|
|
'''
|
|
== Implementation Specification
|
|
(visible only on this page)
|
|
|
|
include::../message.adoc[]
|
|
|
|
include::../highlighting.adoc[]
|
|
|
|
'''
|
|
== Comments And Links
|
|
(visible only on this page)
|
|
|
|
include::../comments-and-links.adoc[]
|
|
|
|
endif::env-github,rspecator-view[]
|