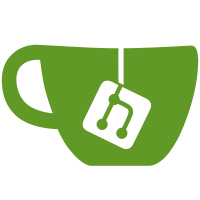
* Revert "Modify rules S2259;S2583;S2589;S3518;S3655;S3959 Remove replacement rules and update description for deprecated SE rules (#4207)" This reverts commit d4d145e532aa487392b1e273e205854f68eb1328. * Revert "SONARJAVA-5102 Deprecate Java SE rules implemented by DBD (#4177)" This reverts commit 952c1cab7b996d1a3e5060bc91745df6543d7eaf.
91 lines
1.9 KiB
Plaintext
91 lines
1.9 KiB
Plaintext
== Why is this an issue?
|
|
|
|
``++Optional++`` value can hold either a value or not. The value held in the ``++Optional++`` can be accessed using the ``++get()++`` method, but it will throw a
|
|
|
|
``++NoSuchElementException++`` if there is no value present. To avoid the exception, calling the ``++isPresent()++`` or ``++! isEmpty()++`` method should always be done before any call to ``++get()++``.
|
|
|
|
|
|
Alternatively, note that other methods such as ``++orElse(...)++``, ``++orElseGet(...)++`` or ``++orElseThrow(...)++`` can be used to specify what to do with an empty ``++Optional++``.
|
|
|
|
=== Noncompliant code example
|
|
|
|
[source,java]
|
|
----
|
|
Optional<String> value = this.getOptionalValue();
|
|
|
|
// ...
|
|
|
|
String stringValue = value.get(); // Noncompliant
|
|
----
|
|
|
|
[source,java]
|
|
----
|
|
if (methodThatReturnsOptional().isEmpty()) {
|
|
throw new NotFoundException();
|
|
}
|
|
String value = methodThatReturnsOptional().get(); // Noncompliant: indirect access, we consider that two consecutive calls can return different values.
|
|
----
|
|
|
|
=== Compliant solution
|
|
|
|
[source,java]
|
|
----
|
|
this.getOptionalValue().ifPresent(stringValue ->
|
|
// Do something with stringValue
|
|
);
|
|
----
|
|
|
|
or
|
|
|
|
[source,java]
|
|
----
|
|
Optional<String> value = this.getOptionalValue();
|
|
|
|
// ...
|
|
|
|
if (value.isPresent()) {
|
|
String stringValue = value.get();
|
|
}
|
|
----
|
|
|
|
or
|
|
|
|
[source,java]
|
|
----
|
|
Optional<String> value = this.getOptionalValue();
|
|
|
|
// ...
|
|
|
|
String stringValue = value.orElse("default");
|
|
----
|
|
|
|
[source,java]
|
|
----
|
|
Optional<String> optional = methodThatReturnsOptional();
|
|
if (optional.isEmpty()) {
|
|
throw new NotFoundException();
|
|
}
|
|
String value = optional.get();
|
|
----
|
|
|
|
include::../see.adoc[]
|
|
|
|
ifdef::env-github,rspecator-view[]
|
|
|
|
'''
|
|
== Implementation Specification
|
|
(visible only on this page)
|
|
|
|
=== Message
|
|
|
|
call "xxx.isPresent()" before accessing the value.
|
|
|
|
|
|
'''
|
|
== Comments And Links
|
|
(visible only on this page)
|
|
|
|
include::../comments-and-links.adoc[]
|
|
|
|
endif::env-github,rspecator-view[]
|