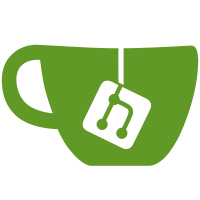
## Review A dedicated reviewer checked the rule description successfully for: - [ ] logical errors and incorrect information - [ ] information gaps and missing content - [ ] text style and tone - [ ] PR summary and labels follow [the guidelines](https://github.com/SonarSource/rspec/#to-modify-an-existing-rule)
83 lines
2.0 KiB
Plaintext
83 lines
2.0 KiB
Plaintext
include::../why-dotnet.adoc[]
|
|
|
|
== How to fix it
|
|
|
|
=== Code examples
|
|
|
|
==== Noncompliant code example
|
|
|
|
`BeginInvoke` without callback:
|
|
|
|
[source,csharp,diff-id=1,diff-type=noncompliant]
|
|
----
|
|
public delegate string AsyncMethodCaller();
|
|
|
|
public static void Main()
|
|
{
|
|
AsyncExample asyncExample = new AsyncExample();
|
|
AsyncMethodCaller caller = new AsyncMethodCaller(asyncExample.MyMethod);
|
|
|
|
// Initiate the asynchronous call.
|
|
IAsyncResult result = caller.BeginInvoke(null, null); // Noncompliant: not paired with EndInvoke
|
|
}
|
|
----
|
|
|
|
`BeginInvoke` with callback:
|
|
|
|
[source,csharp,diff-id=2,diff-type=noncompliant]
|
|
----
|
|
public delegate string AsyncMethodCaller();
|
|
|
|
public static void Main()
|
|
{
|
|
AsyncExample asyncExample = new AsyncExample();
|
|
AsyncMethodCaller caller = new AsyncMethodCaller(asyncExample.MyMethod);
|
|
|
|
IAsyncResult result = caller.BeginInvoke(
|
|
new AsyncCallback((IAsyncResult ar) => {}),
|
|
null); // Noncompliant: not paired with EndInvoke
|
|
}
|
|
----
|
|
|
|
==== Compliant solution
|
|
|
|
`BeginInvoke` without callback:
|
|
|
|
[source,csharp,diff-id=1,diff-type=compliant]
|
|
----
|
|
public delegate string AsyncMethodCaller();
|
|
|
|
public static void Main()
|
|
{
|
|
AsyncExample asyncExample = new AsyncExample();
|
|
AsyncMethodCaller caller = new AsyncMethodCaller(asyncExample.MyMethod);
|
|
|
|
IAsyncResult result = caller.BeginInvoke(null, null);
|
|
|
|
string returnValue = caller.EndInvoke(result);
|
|
}
|
|
----
|
|
|
|
`BeginInvoke` with callback:
|
|
|
|
[source,csharp,diff-id=2,diff-type=compliant]
|
|
----
|
|
public delegate string AsyncMethodCaller();
|
|
|
|
public static void Main()
|
|
{
|
|
AsyncExample asyncExample = new AsyncExample();
|
|
AsyncMethodCaller caller = new AsyncMethodCaller(asyncExample.MyMethod);
|
|
|
|
IAsyncResult result = caller.BeginInvoke(
|
|
new AsyncCallback((IAsyncResult ar) =>
|
|
{
|
|
// Call EndInvoke to retrieve the results.
|
|
string returnValue = caller.EndInvoke(ar);
|
|
}), null);
|
|
}
|
|
----
|
|
|
|
include::../resources-dotnet.adoc[]
|
|
|
|
include::../rspecator.adoc[] |