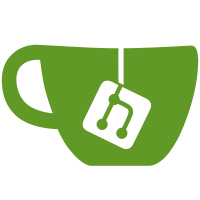
When an include is not surrounded by empty lines, its content is inlined on the same line as the adjacent content. That can lead to broken tags and other display issues. This PR fixes all such includes and introduces a validation step that forbids introducing the same problem again.
68 lines
2.3 KiB
Plaintext
68 lines
2.3 KiB
Plaintext
Predefined permissions, also known as https://docs.aws.amazon.com/AmazonS3/latest/userguide/acl-overview.html#canned-acl[canned ACLs], are an easy way to grant large privileges to predefined groups or users.
|
|
|
|
The following canned ACLs are security-sensitive:
|
|
|
|
* `PUBLIC_READ`, `PUBLIC_READ_WRITE` grant respectively "read" and "read and write" privileges to anyone, either authenticated or anonymous (`AllUsers` group).
|
|
* `AUTHENTICATED_READ` grants "read" privilege to all authenticated users (`AuthenticatedUsers` group).
|
|
|
|
|
|
include::../ask-yourself.adoc[]
|
|
|
|
== Recommended Secure Coding Practices
|
|
|
|
It's recommended to implement the least privilege policy, i.e., to only grant users the necessary permissions for their required tasks. In the context of canned ACL, set it to `PRIVATE` (the default one), and if needed more granularity then use an appropriate S3 policy.
|
|
|
|
|
|
== Sensitive Code Example
|
|
|
|
All users, either authenticated or anonymous, have read and write permissions with the `PUBLIC_READ_WRITE` access control:
|
|
|
|
[source,javascript]
|
|
----
|
|
const s3 = require('aws-cdk-lib/aws-s3');
|
|
|
|
new s3.Bucket(this, 'bucket', {
|
|
accessControl: s3.BucketAccessControl.PUBLIC_READ_WRITE // Sensitive
|
|
});
|
|
|
|
new s3deploy.BucketDeployment(this, 'DeployWebsite', {
|
|
accessControl: s3.BucketAccessControl.PUBLIC_READ_WRITE // Sensitive
|
|
});
|
|
----
|
|
|
|
== Compliant Solution
|
|
|
|
With the `PRIVATE` access control (default), only the bucket owner has the read/write permissions on the bucket and its ACL.
|
|
|
|
[source,javascript]
|
|
----
|
|
const s3 = require('aws-cdk-lib/aws-s3');
|
|
|
|
new s3.Bucket(this, 'bucket', {
|
|
accessControl: s3.BucketAccessControl.PRIVATE
|
|
});
|
|
|
|
new s3deploy.BucketDeployment(this, 'DeployWebsite', {
|
|
accessControl: s3.BucketAccessControl.PRIVATE
|
|
});
|
|
----
|
|
|
|
include::../see.adoc[]
|
|
|
|
* https://docs.aws.amazon.com/cdk/api/v2/docs/aws-cdk-lib.aws_s3.Bucket.html[AWS CDK version 2] - Class Bucket (construct)
|
|
ifdef::env-github,rspecator-view[]
|
|
|
|
'''
|
|
== Implementation Specification
|
|
(visible only on this page)
|
|
|
|
=== Message
|
|
|
|
* Primary: Make sure granting [PUBLIC_READ|PUBLIC_READ_WRITE|AUTHENTICATED_READ] access is safe here.
|
|
* Secondary: Propagated setting.
|
|
|
|
* Primary: Make sure allowing unrestricted access to objects from this bucket is safe here.
|
|
* Secondary: Propagated setting.
|
|
|
|
endif::env-github,rspecator-view[]
|