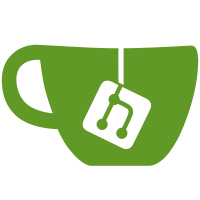
Update all links to C++ Core Guidelines to `e49158a`.
Refresh done using the following script and some manual edits:
db76e34e74/personal/fred-tingaud/rspec/refresh-cppcoreguidelines.py
When re-using this script, be mindful that:
- it does not cover `shared_content`
- it does not properly escape inline code in links (e.g., "[=]" or "`mutex`es")
- it does not change `C++` to `{cpp}` in link titles.
Co-authored-by: Marco Borgeaud <marco.borgeaud@sonarsource.com>
58 lines
1.7 KiB
Plaintext
58 lines
1.7 KiB
Plaintext
== Why is this an issue?
|
|
|
|
If the lifetime of the arguments passed to a lambda function is longer than the lifetime of the lambda itself, these arguments can be passed by reference.
|
|
|
|
Doing so avoids copying potentially big objects and it should be preferred over using copy capture.
|
|
|
|
|
|
This rule reports an issue if a lambda passed into an algorithm that uses it locally (all algorithms in headers ``++<algorithm>++`` and ``++<numeric>++``) captures some values.
|
|
|
|
|
|
=== Noncompliant code example
|
|
|
|
[source,cpp]
|
|
----
|
|
using Vec = vector<int>;
|
|
|
|
Vec applyPermutation(const Vec& v, const Vec& permutation) {
|
|
assert(v.size() == permutation.size());
|
|
|
|
const auto n = v.size();
|
|
Vec result(n);
|
|
|
|
// Noncompliant: this will copy the entire v vector for each iteration, resulting in n^2 operations
|
|
transform(permutation.begin(), permutation.end(), back_inserter(result),
|
|
[v](int position){ return v[position]; });
|
|
|
|
return result;
|
|
}
|
|
----
|
|
|
|
|
|
=== Compliant solution
|
|
|
|
[source,cpp]
|
|
----
|
|
using Vec = vector<int>;
|
|
|
|
Vec applyPermutation(const Vec& v, const Vec& permutation) {
|
|
assert(v.size() == permutation.size());
|
|
|
|
const auto n = v.size();
|
|
Vec result(n);
|
|
|
|
// Compliant: this will NOT copy the entire v vector for each iteration, resulting in n operations
|
|
transform(permutation.begin(), permutation.end(), back_inserter(result),
|
|
[&v](int position){ return v[position]; });
|
|
|
|
return result;
|
|
}
|
|
----
|
|
|
|
|
|
== Resources
|
|
|
|
* {cpp} Core Guidelines - https://github.com/isocpp/CppCoreGuidelines/blob/e49158a/CppCoreGuidelines.md#f52-prefer-capturing-by-reference-in-lambdas-that-will-be-used-locally-including-passed-to-algorithms[F.52: Prefer capturing by reference in lambdas that will be used locally, including passed to algorithms]
|
|
|
|
|