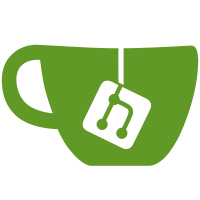
When an include is not surrounded by empty lines, its content is inlined on the same line as the adjacent content. That can lead to broken tags and other display issues. This PR fixes all such includes and introduces a validation step that forbids introducing the same problem again.
150 lines
3.7 KiB
Plaintext
150 lines
3.7 KiB
Plaintext
== Why is this an issue?
|
|
|
|
include::../description.adoc[]
|
|
|
|
=== Noncompliant code example
|
|
|
|
In a Symfony web application:
|
|
|
|
* the ``++vote++`` method of a https://symfony.com/doc/current/security/voters.html[VoterInterface] type is not compliant when it returns only an affirmative decision (``++ACCESS_GRANTED++``):
|
|
|
|
[source,php]
|
|
----
|
|
class NoncompliantVoterInterface implements VoterInterface
|
|
{
|
|
public function vote(TokenInterface $token, $subject, array $attributes)
|
|
{
|
|
return self::ACCESS_GRANTED; // Noncompliant
|
|
}
|
|
}
|
|
----
|
|
|
|
* the ``++voteOnAttribute++`` method of a https://symfony.com/doc/current/security/voters.html[Voter] type is not compliant when it returns only an affirmative decision (``++true++``):
|
|
|
|
[source,php]
|
|
----
|
|
class NoncompliantVoter extends Voter
|
|
{
|
|
protected function supports(string $attribute, $subject)
|
|
{
|
|
return true;
|
|
}
|
|
|
|
protected function voteOnAttribute(string $attribute, $subject, TokenInterface $token)
|
|
{
|
|
return true; // Noncompliant
|
|
}
|
|
}
|
|
----
|
|
|
|
In a Laravel web application:
|
|
|
|
* the ``++define++``, ``++before++``, and ``++after++`` methods of a https://laravel.com/docs/8.x/authorization[Gate] are not compliant when they return only an affirmative decision (``++true++`` or ``++Response::allow()++``):
|
|
|
|
[source,php]
|
|
----
|
|
class NoncompliantGuard
|
|
{
|
|
public function boot()
|
|
{
|
|
Gate::define('xxx', function ($user) {
|
|
return true; // Noncompliant
|
|
});
|
|
|
|
Gate::define('xxx', function ($user) {
|
|
return Response::allow(); // Noncompliant
|
|
});
|
|
}
|
|
}
|
|
----
|
|
|
|
=== Compliant solution
|
|
|
|
In a Symfony web application:
|
|
|
|
* the ``++vote++`` method of a https://symfony.com/doc/current/security/voters.html[VoterInterface] type should return a negative decision (``++ACCESS_DENIED++``) or abstain from making a decision (``++ACCESS_ABSTAIN++``):
|
|
|
|
[source,php]
|
|
----
|
|
class CompliantVoterInterface implements VoterInterface
|
|
{
|
|
public function vote(TokenInterface $token, $subject, array $attributes)
|
|
{
|
|
if (foo()) {
|
|
return self::ACCESS_GRANTED; // Compliant
|
|
} else if (bar()) {
|
|
return self::ACCESS_ABSTAIN;
|
|
}
|
|
return self::ACCESS_DENIED;
|
|
}
|
|
}
|
|
----
|
|
|
|
* the ``++voteOnAttribute++`` method of a https://symfony.com/doc/current/security/voters.html[Voter] type should return a negative decision (``++false++``):
|
|
|
|
[source,php]
|
|
----
|
|
class CompliantVoter extends Voter
|
|
{
|
|
protected function supports(string $attribute, $subject)
|
|
{
|
|
return true;
|
|
}
|
|
|
|
protected function voteOnAttribute(string $attribute, $subject, TokenInterface $token)
|
|
{
|
|
if (foo()) {
|
|
return true; // Compliant
|
|
}
|
|
return false;
|
|
}
|
|
}
|
|
----
|
|
|
|
In a Laravel web application:
|
|
|
|
* the ``++define++``, ``++before++``, and ``++after++`` methods of a https://laravel.com/docs/8.x/authorization[Gate] should return a negative decision (``++false++`` or ``++Response::deny()++``) or abstain from making a decision (``++null++``):
|
|
|
|
[source,php]
|
|
----
|
|
class NoncompliantGuard
|
|
{
|
|
public function boot()
|
|
{
|
|
Gate::define('xxx', function ($user) {
|
|
if (foo()) {
|
|
return true; // Compliant
|
|
}
|
|
return false;
|
|
});
|
|
|
|
Gate::define('xxx', function ($user) {
|
|
if (foo()) {
|
|
return Response::allow(); // Compliant
|
|
}
|
|
return Response::deny();
|
|
});
|
|
}
|
|
}
|
|
----
|
|
|
|
include::../see.adoc[]
|
|
|
|
ifdef::env-github,rspecator-view[]
|
|
|
|
'''
|
|
== Implementation Specification
|
|
(visible only on this page)
|
|
|
|
=== Message
|
|
|
|
Vote methods should return at least once a negative response
|
|
|
|
|
|
=== Highlighting
|
|
|
|
\[vote|voteOnAttribute|Gate::define|Gate::before|Gate::after] method name
|
|
|
|
|
|
endif::env-github,rspecator-view[]
|