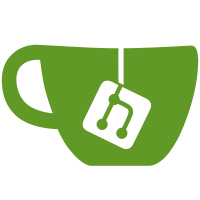
Inline adoc files when they are included exactly once. Also fix language tags because this inlining gives us better information on what language the code is written in.
95 lines
2.1 KiB
Plaintext
95 lines
2.1 KiB
Plaintext
== Why is this an issue?
|
|
|
|
Java 7 introduced the try-with-resources statement, which guarantees that the resource in question will be closed. Since the new syntax is closer to bullet-proof, it should be preferred over the older ``++try++``/``++catch++``/``++finally++`` version.
|
|
|
|
|
|
This rule checks that ``++close++``-able resources are opened in a try-with-resources statement.
|
|
|
|
|
|
*Note* that this rule is automatically disabled when the project's ``++sonar.java.source++`` is lower than ``++7++``.
|
|
|
|
|
|
=== Noncompliant code example
|
|
|
|
[source,java]
|
|
----
|
|
FileReader fr = null;
|
|
BufferedReader br = null;
|
|
try {
|
|
fr = new FileReader(fileName);
|
|
br = new BufferedReader(fr);
|
|
return br.readLine();
|
|
} catch (...) {
|
|
} finally {
|
|
if (br != null) {
|
|
try {
|
|
br.close();
|
|
} catch(IOException e){...}
|
|
}
|
|
if (fr != null ) {
|
|
try {
|
|
br.close();
|
|
} catch(IOException e){...}
|
|
}
|
|
}
|
|
----
|
|
|
|
|
|
=== Compliant solution
|
|
|
|
[source,java]
|
|
----
|
|
try (
|
|
FileReader fr = new FileReader(fileName);
|
|
BufferedReader br = new BufferedReader(fr)
|
|
) {
|
|
return br.readLine();
|
|
}
|
|
catch (...) {}
|
|
----
|
|
or
|
|
|
|
[source,java]
|
|
----
|
|
try (BufferedReader br =
|
|
new BufferedReader(new FileReader(fileName))) { // no need to name intermediate resources if you don't want to
|
|
return br.readLine();
|
|
}
|
|
catch (...) {}
|
|
----
|
|
|
|
|
|
== Resources
|
|
|
|
* https://wiki.sei.cmu.edu/confluence/x/6DZGBQ[CERT, ERR54-J.] - Use a try-with-resources statement to safely handle closeable resources
|
|
|
|
|
|
ifdef::env-github,rspecator-view[]
|
|
|
|
'''
|
|
== Implementation Specification
|
|
(visible only on this page)
|
|
|
|
=== Message
|
|
|
|
Change this "try" to a try-with-resources.
|
|
|
|
|
|
'''
|
|
== Comments And Links
|
|
(visible only on this page)
|
|
|
|
=== on 12 Oct 2014, 18:26:26 Freddy Mallet wrote:
|
|
Minor point @Ann but I would associate the tag 'bug' to this rule.
|
|
|
|
=== on 12 Oct 2014, 22:18:37 Ann Campbell wrote:
|
|
I disagree [~freddy.mallet]. Properly written (and we have other rules to catch if it's not) there's no bug.
|
|
|
|
=== on 17 Oct 2014, 10:17:50 Freddy Mallet wrote:
|
|
Ok [~ann.campbell.2]
|
|
|
|
=== on 6 Jan 2016, 10:16:15 Nicolas Peru wrote:
|
|
Adding to default profile as we can rely on detection of java version.
|
|
|
|
endif::env-github,rspecator-view[]
|