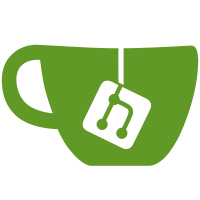
Inline adoc files when they are included exactly once. Also fix language tags because this inlining gives us better information on what language the code is written in.
95 lines
1.6 KiB
Plaintext
95 lines
1.6 KiB
Plaintext
== Why is this an issue?
|
|
|
|
include::../description-dotnet.adoc[]
|
|
|
|
=== Noncompliant code example
|
|
|
|
[source,csharp]
|
|
----
|
|
class MyClass
|
|
{
|
|
private object obj = new object();
|
|
|
|
public void DoSomethingWithMonitor()
|
|
{
|
|
Monitor.Enter(obj); // Noncompliant
|
|
if (IsInitialized())
|
|
{
|
|
// ...
|
|
Monitor.Exit(obj);
|
|
}
|
|
}
|
|
|
|
private ReaderWriterLockSlim lockObj = new ReaderWriterLockSlim();
|
|
|
|
public void DoSomethingWithReaderWriteLockSlim()
|
|
{
|
|
lockObj.EnterReadLock(); // Noncompliant
|
|
if (IsInitialized())
|
|
{
|
|
// ...
|
|
lockObj.ExitReadLock();
|
|
}
|
|
}
|
|
}
|
|
----
|
|
|
|
|
|
=== Compliant solution
|
|
|
|
[source,csharp]
|
|
----
|
|
class MyClass
|
|
{
|
|
private object obj = new object();
|
|
|
|
public void DoSomethingWithMonitor()
|
|
{
|
|
lock(obj) // lock() {...} is easier to use than explicit Monitor calls
|
|
{
|
|
if (IsInitialized())
|
|
{
|
|
}
|
|
}
|
|
}
|
|
|
|
private ReaderWriterLockSlim lockObj = new ReaderWriterLockSlim();
|
|
|
|
public void DoSomethingWithReaderWriteLockSlim()
|
|
{
|
|
lockObj.EnterReadLock();
|
|
try
|
|
{
|
|
if (IsInitialized())
|
|
{
|
|
}
|
|
}
|
|
finally
|
|
{
|
|
lockObj.ExitReadLock();
|
|
}
|
|
}
|
|
}
|
|
----
|
|
|
|
|
|
include::../see.adoc[]
|
|
|
|
* https://docs.microsoft.com/en-us/dotnet/standard/threading/overview-of-synchronization-primitives#synchronization-of-access-to-a-shared-resource[Synchronization of access to a shared resource].
|
|
|
|
|
|
ifdef::env-github,rspecator-view[]
|
|
|
|
'''
|
|
== Implementation Specification
|
|
(visible only on this page)
|
|
|
|
include::../message.adoc[]
|
|
|
|
'''
|
|
== Comments And Links
|
|
(visible only on this page)
|
|
|
|
include::../comments-and-links.adoc[]
|
|
endif::env-github,rspecator-view[]
|