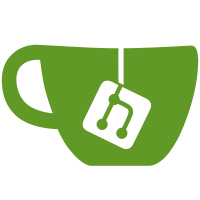
* SONARKT-569 Modify rule S4830: add support for WebViews * Fix list of allowed frameworks * Add Google Support link * Have non-compliant and compliant code examples next to each other and in diff * Update rules/S4830/kotlin/how-to-fix-it/android-webview.adoc Co-authored-by: Egon Okerman <egon.okerman@sonarsource.com> * Update rules/S4830/kotlin/how-to-fix-it/android-webview.adoc Co-authored-by: Egon Okerman <egon.okerman@sonarsource.com> --------- Co-authored-by: Egon Okerman <egon.okerman@sonarsource.com>
45 lines
1.5 KiB
Plaintext
45 lines
1.5 KiB
Plaintext
== How to fix it in Android WebView
|
|
|
|
=== Code examples
|
|
|
|
include::../../common/fix/code-rationale.adoc[]
|
|
|
|
The certificate validation gets disabled by overriding the `onReceivedSslError` method of the `WebViewClient` class with an implementation that calls `SslErrorHandler.proceed()` unconditionally, and that never calls `SslErrorHandler.cancel()`.
|
|
|
|
This means that a certificate initially rejected by the system will be accepted by the `WebViewClient`, regardless of its origin.
|
|
|
|
==== Noncompliant code example
|
|
|
|
[source,kotlin,diff-id=101,diff-type=noncompliant]
|
|
----
|
|
class MyWebViewClient : WebViewClient() {
|
|
override fun onReceivedSslError(view: WebView, handler: SslErrorHandler, error: SslError) =
|
|
handler.proceed() // Noncompliant
|
|
}
|
|
----
|
|
|
|
==== Compliant solution
|
|
|
|
You need to implement a validation of the server certificate received in the `SslErrorHandler` object, calling `proceed` and `cancel` appropriately.
|
|
|
|
[source,kotlin,diff-id=101,diff-type=compliant]
|
|
----
|
|
class MyWebViewClient : WebViewClient() {
|
|
override fun onReceivedSslError(view: WebView, handler: SslErrorHandler, error: SslError) {
|
|
if (error.certificate.isServerCertificateValid()) {
|
|
handler.proceed()
|
|
} else {
|
|
handler.cancel()
|
|
}
|
|
}
|
|
|
|
private fun SslCertificate.isServerCertificateValid(): Boolean {
|
|
// Implement the server certificate validation logic here ...
|
|
}
|
|
}
|
|
----
|
|
|
|
=== How does this work?
|
|
|
|
include::../../common/fix/validation.adoc[]
|