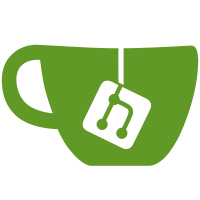
## Review A dedicated reviewer checked the rule description successfully for: - [ ] logical errors and incorrect information - [ ] information gaps and missing content - [ ] text style and tone - [ ] PR summary and labels follow [the guidelines](https://github.com/SonarSource/rspec/#to-modify-an-existing-rule)
40 lines
1.1 KiB
Plaintext
40 lines
1.1 KiB
Plaintext
== How to fix it in BouncyCastle
|
|
|
|
=== Code examples
|
|
|
|
The example uses a hardcoded IV as a nonce, which causes AES-CCM to be insecure. To fix it, a nonce is randomly generated instead.
|
|
|
|
==== Noncompliant code example
|
|
|
|
[source,java,diff-id=201,diff-type=noncompliant]
|
|
----
|
|
public void encrypt(byte[] key, byte[] ptxt) {
|
|
byte[] nonce = "7cVgr5cbdCZV".getBytes(StandardCharsets.UTF_8);
|
|
|
|
BlockCipher engine = new AESEngine();
|
|
AEADParameters params = new AEADParameters(new KeyParameter(key), 128, nonce);
|
|
CCMBlockCipher cipher = new CCMBlockCipher(engine);
|
|
|
|
cipher.init(true, params); // Noncompliant
|
|
}
|
|
----
|
|
|
|
==== Compliant solution
|
|
|
|
[source,java,diff-id=201,diff-type=compliant]
|
|
----
|
|
public void encrypt(byte[] key, byte[] ptxt) {
|
|
SecureRandom random = new SecureRandom();
|
|
byte[] nonce = new byte[12];
|
|
random.nextBytes(nonce);
|
|
|
|
BlockCipher engine = new AESEngine();
|
|
AEADParameters params = new AEADParameters(new KeyParameter(key), 128, nonce);
|
|
CCMBlockCipher cipher = new CCMBlockCipher(engine);
|
|
|
|
cipher.init(true, params);
|
|
}
|
|
----
|
|
|
|
include::../../common/how-does-this-work.adoc[]
|