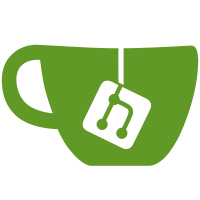
Inline adoc files when they are included exactly once. Also fix language tags because this inlining gives us better information on what language the code is written in.
77 lines
1.8 KiB
Plaintext
77 lines
1.8 KiB
Plaintext
== Why is this an issue?
|
|
|
|
In Java 16 records represent a brief notation for immutable data structures. Records have autogenerated implementations for constructors with all parameters, ``++getters++``, ``++equals++``, ``++hashcode++`` and ``++toString++``. Although these methods can still be overridden inside records, there is no use to do so if no special logic is required.
|
|
|
|
|
|
This rule reports an issue on empty compact constructors, trivial canonical constructors and simple getter methods with no additional logic.
|
|
|
|
|
|
=== Noncompliant code example
|
|
|
|
[source,java]
|
|
----
|
|
record Person(String name, int age) {
|
|
Person(String name, int age) { // Noncompliant, already autogenerated
|
|
this.name = name;
|
|
this.age = age;
|
|
}
|
|
}
|
|
|
|
record Person(String name, int age) {
|
|
Person { // Noncompliant, no need for empty compact constructor
|
|
}
|
|
public String name() { // Noncompliant, already autogenerated
|
|
return name;
|
|
}
|
|
}
|
|
----
|
|
|
|
|
|
=== Compliant solution
|
|
|
|
[source,java]
|
|
----
|
|
record Person(String name, int age) { } // Compliant
|
|
|
|
record Person(String name, int age) {
|
|
Person(String name, int age) { // Compliant
|
|
this.name = name.toLowerCase(Locale.ROOT);
|
|
this.age = age;
|
|
}
|
|
}
|
|
|
|
record Person(String name, int age) {
|
|
Person { // Compliant
|
|
if (age < 0) {
|
|
throw new IllegalArgumentException("Negative age");
|
|
}
|
|
}
|
|
public String name() { // Compliant
|
|
return name.toUpperCase(Locale.ROOT);
|
|
}
|
|
}
|
|
----
|
|
|
|
|
|
== Resources
|
|
|
|
* https://docs.oracle.com/javase/specs/jls/se16/html/jls-8.html#jls-8.10[Records specification]
|
|
|
|
ifdef::env-github,rspecator-view[]
|
|
|
|
'''
|
|
== Implementation Specification
|
|
(visible only on this page)
|
|
|
|
=== Message
|
|
|
|
Remove this redundant constructor/method which is the same as a default one
|
|
|
|
|
|
=== Highlighting
|
|
|
|
method name
|
|
|
|
|
|
endif::env-github,rspecator-view[]
|