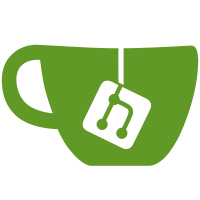
When an include is not surrounded by empty lines, its content is inlined on the same line as the adjacent content. That can lead to broken tags and other display issues. This PR fixes all such includes and introduces a validation step that forbids introducing the same problem again.
71 lines
1.4 KiB
Plaintext
71 lines
1.4 KiB
Plaintext
== Why is this an issue?
|
|
|
|
Needing to cast from an ``++interface++`` to a concrete type indicates that something is wrong with the abstractions in use, likely that something is missing from the ``++interface++``. Instead of casting to a discrete type, the missing functionality should be added to the ``++interface++``. Otherwise there is a risk of runtime exceptions.
|
|
|
|
|
|
=== Noncompliant code example
|
|
|
|
[source,csharp]
|
|
----
|
|
public interface IMyInterface
|
|
{
|
|
void DoStuff();
|
|
}
|
|
|
|
public class MyClass1 : IMyInterface
|
|
{
|
|
public int Data { get { return new Random().Next(); } }
|
|
|
|
public void DoStuff()
|
|
{
|
|
// TODO...
|
|
}
|
|
}
|
|
|
|
public static class DowncastExampleProgram
|
|
{
|
|
static void EntryPoint(IMyInterface interfaceRef)
|
|
{
|
|
MyClass1 class1 = (MyClass1)interfaceRef; // Noncompliant
|
|
int privateData = class1.Data;
|
|
|
|
class1 = interfaceRef as MyClass1; // Noncompliant
|
|
if (class1 != null)
|
|
{
|
|
// ...
|
|
}
|
|
}
|
|
}
|
|
----
|
|
|
|
|
|
=== Exceptions
|
|
|
|
Casting to ``++object++`` doesn't raise an issue, because it can never fail.
|
|
|
|
[source,csharp]
|
|
----
|
|
static void EntryPoint(IMyInterface interfaceRef)
|
|
{
|
|
var o = (object)interfaceRef;
|
|
...
|
|
}
|
|
----
|
|
|
|
|
|
ifdef::env-github,rspecator-view[]
|
|
|
|
'''
|
|
== Implementation Specification
|
|
(visible only on this page)
|
|
|
|
include::../message.adoc[]
|
|
|
|
'''
|
|
== Comments And Links
|
|
(visible only on this page)
|
|
|
|
include::../comments-and-links.adoc[]
|
|
|
|
endif::env-github,rspecator-view[]
|