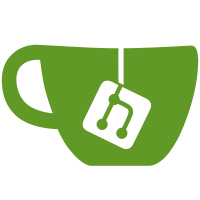
Inline adoc files when they are included exactly once. Also fix language tags because this inlining gives us better information on what language the code is written in.
67 lines
1.4 KiB
Plaintext
67 lines
1.4 KiB
Plaintext
== Why is this an issue?
|
|
|
|
A ``++std::optional<T>++`` can contain a value of type ``++T++``, or can be empty. So in order to avoid crashes or misbehaviors of code, it is better to check the presence of a value in an optional before accessing it. This can be done:
|
|
|
|
* By using the ``++has_value++`` member function
|
|
* By converting the optional to a boolean
|
|
|
|
Alternatively, the member function ``++value_or++`` can be used to return the contained value or a specific value if the optional is empty.
|
|
|
|
|
|
=== Noncompliant code example
|
|
|
|
[source,cpp]
|
|
----
|
|
using namespace std;
|
|
|
|
auto create(bool b) {
|
|
return b ? optional<string>{"myString"} : nullopt;
|
|
}
|
|
|
|
void f(bool b) {
|
|
auto str = create(b);
|
|
cout << *str << endl; // Noncompliant, optional could be empty
|
|
}
|
|
----
|
|
|
|
|
|
=== Compliant solution
|
|
|
|
[source,cpp]
|
|
----
|
|
using namespace std;
|
|
|
|
auto create(bool b) {
|
|
return b ? optional<string>{"myString"} : nullopt;
|
|
}
|
|
|
|
void f1(bool b) {
|
|
auto str = create(b);
|
|
if (str.hasValue()) {
|
|
cout << *str << endl;
|
|
}
|
|
}
|
|
void f2(bool b) {
|
|
if (auto str = create(b)) {
|
|
cout << *str << endl;
|
|
}
|
|
}
|
|
void f3(bool b) {
|
|
auto str = create(b);
|
|
cout << str.value_or("<empty>") << endl;
|
|
}
|
|
----
|
|
|
|
ifdef::env-github,rspecator-view[]
|
|
|
|
'''
|
|
== Implementation Specification
|
|
(visible only on this page)
|
|
|
|
=== Message
|
|
|
|
Check this optional contains a value before accessing it.
|
|
|
|
|
|
endif::env-github,rspecator-view[]
|