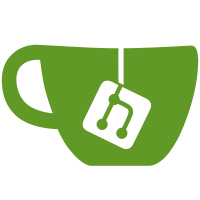
Inline adoc files when they are included exactly once. Also fix language tags because this inlining gives us better information on what language the code is written in.
78 lines
1.6 KiB
Plaintext
78 lines
1.6 KiB
Plaintext
== Why is this an issue?
|
|
|
|
When the names of arguments in a function call match the names of the function parameters, it contributes to clearer, more readable code. However, when the names match, but are passed in a different order than the function parameters, it indicates a mistake in the parameter order which will likely lead to unexpected results.
|
|
|
|
=== Noncompliant code example
|
|
|
|
[source,javascript]
|
|
----
|
|
function divide(divisor, dividend) {
|
|
return divisor/dividend;
|
|
}
|
|
|
|
function doTheThing() {
|
|
var divisor = 15;
|
|
var dividend = 5;
|
|
|
|
var result = divide(dividend, divisor); // Noncompliant; operation succeeds, but result is unexpected
|
|
//...
|
|
}
|
|
----
|
|
|
|
=== Compliant solution
|
|
|
|
[source,javascript]
|
|
----
|
|
function divide(divisor, dividend) {
|
|
return divisor/dividend;
|
|
}
|
|
|
|
function doTheThing() {
|
|
var divisor = 15;
|
|
var dividend = 5;
|
|
|
|
var result = divide(divisor, dividend);
|
|
//...
|
|
}
|
|
----
|
|
|
|
=== Exceptions
|
|
|
|
Swapped arguments that are compared beforehand in an enclosing ``++if++``-statement are ignored:
|
|
|
|
[source,javascript]
|
|
----
|
|
function divide(divisor, dividend) {
|
|
return divisor/dividend;
|
|
}
|
|
|
|
function doTheThing() {
|
|
var divisor = 15;
|
|
var dividend = 5;
|
|
if (divisor > dividend) {
|
|
var result = divide(dividend, divisor);
|
|
//...
|
|
}
|
|
}
|
|
----
|
|
|
|
ifdef::env-github,rspecator-view[]
|
|
|
|
'''
|
|
== Implementation Specification
|
|
(visible only on this page)
|
|
|
|
=== Message
|
|
|
|
Arguments to "xxx" have the same names but not the same order as the function parameters.
|
|
|
|
|
|
include::../highlighting.adoc[]
|
|
|
|
'''
|
|
== Comments And Links
|
|
(visible only on this page)
|
|
|
|
include::../comments-and-links.adoc[]
|
|
endif::env-github,rspecator-view[]
|