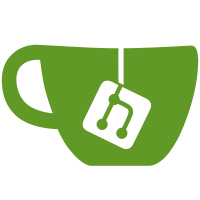
Inline adoc files when they are included exactly once. Also fix language tags because this inlining gives us better information on what language the code is written in.
62 lines
1.3 KiB
Plaintext
62 lines
1.3 KiB
Plaintext
== Why is this an issue?
|
|
|
|
Nullable value types can hold either a value or `null`. The value held in the nullable type can be accessed with the `Value` property or by casting it to the underlying type. Still, both operations throw an `InvalidOperationException` when the value is `null`. A nullable type should always be tested before the value is accessed to avoid the exception.
|
|
|
|
|
|
=== Noncompliant code example
|
|
|
|
[source,csharp]
|
|
----
|
|
public void Sample(bool condition)
|
|
{
|
|
int? nullableValue = condition ? 42 : null;
|
|
Console.WriteLine(nullableValue.Value); // Noncompliant
|
|
|
|
int? nullableCast = condition ? 42 : null;
|
|
Console.WriteLine((int)nullableCast); // Noncompliant
|
|
}
|
|
----
|
|
|
|
|
|
=== Compliant solution
|
|
|
|
[source,csharp]
|
|
----
|
|
public void Sample(bool condition)
|
|
{
|
|
int? nullableValue = condition ? 42 : null;
|
|
if (nullableValue.HasValue)
|
|
{
|
|
Console.WriteLine(nullableValue.Value);
|
|
}
|
|
|
|
int? nullableCast = condition ? 42 : null;
|
|
if (nullableCast is not null)
|
|
{
|
|
Console.WriteLine((int)nullableCast);
|
|
}
|
|
}
|
|
----
|
|
|
|
|
|
include::../see.adoc[]
|
|
|
|
|
|
ifdef::env-github,rspecator-view[]
|
|
|
|
'''
|
|
== Implementation Specification
|
|
(visible only on this page)
|
|
|
|
=== Message
|
|
|
|
"xxx" is null on at least one execution path.
|
|
|
|
|
|
'''
|
|
== Comments And Links
|
|
(visible only on this page)
|
|
|
|
include::../comments-and-links.adoc[]
|
|
endif::env-github,rspecator-view[]
|