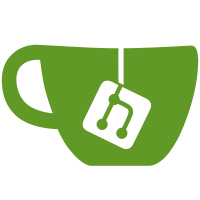
Improvement identified in #2790. Add a prefix to the diff-id when it is used multiple times in different "how to fix it in XYZ" sections to avoid ambiguity and pedantically follow the spec: > A single and unique diff-id should be used only once for each type of code example as shown in the description of a rule. Obvious typos around `diff-type` were fixed.
56 lines
1.2 KiB
Plaintext
56 lines
1.2 KiB
Plaintext
== How to fix it in Sequelize
|
|
|
|
=== Code examples
|
|
|
|
include::../../common/fix/code-rationale.adoc[]
|
|
|
|
==== Noncompliant code example
|
|
|
|
[source,javascript,diff-id=11,diff-type=noncompliant]
|
|
----
|
|
async function index(req, res) {
|
|
const { db, User } = req.app.get('sequelize');
|
|
|
|
let loggedInUser = await db.query(
|
|
`SELECT * FROM users WHERE user = '${req.query.user}' AND pass = '${req.query.pass}'`,
|
|
{
|
|
model: User,
|
|
}
|
|
); // Noncompliant
|
|
|
|
res.send(JSON.stringify(loggedInUser));
|
|
res.end();
|
|
}}
|
|
----
|
|
|
|
==== Compliant solution
|
|
|
|
[source,javascript,diff-id=11,diff-type=compliant]
|
|
----
|
|
async function index(req, res) {
|
|
const { db, User, QueryTypes } = req.app.get('sequelize');
|
|
|
|
let user = req.query.user;
|
|
let pass = req.query.pass;
|
|
|
|
let loggedInUser = await db.query(
|
|
`SELECT * FROM users WHERE user = $user AND pass = $pass`,
|
|
{
|
|
bind: {
|
|
user: user,
|
|
pass: pass,
|
|
},
|
|
type: QueryTypes.SELECT,
|
|
model: User,
|
|
}
|
|
);
|
|
|
|
res.send(JSON.stringify(loggedInUser));
|
|
res.end();
|
|
}
|
|
----
|
|
|
|
=== How does this work?
|
|
|
|
include::../../common/fix/prepared-statements.adoc[]
|