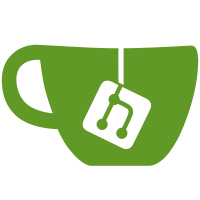
Inline adoc files when they are included exactly once. Also fix language tags because this inlining gives us better information on what language the code is written in.
85 lines
1.5 KiB
Plaintext
85 lines
1.5 KiB
Plaintext
== Why is this an issue?
|
|
|
|
Exceptions types should provide the following constructors:
|
|
|
|
* ``++public MyException()++``
|
|
* ``++public MyException(string)++``
|
|
* ``++public MyException(string, Exception)++``
|
|
* ``++protected++`` or ``++private MyException(SerializationInfo, StreamingContext)++``
|
|
|
|
That fourth constructor should be ``++protected++`` in unsealed classes, and ``++private++`` in sealed classes.
|
|
|
|
|
|
Not having this full set of constructors can make it difficult to handle exceptions.
|
|
|
|
|
|
=== Noncompliant code example
|
|
|
|
[source,csharp]
|
|
----
|
|
using System;
|
|
|
|
namespace MyLibrary
|
|
{
|
|
public class MyException // Noncompliant: several constructors are missing
|
|
{
|
|
public MyException()
|
|
{
|
|
}
|
|
}
|
|
}
|
|
----
|
|
|
|
|
|
=== Compliant solution
|
|
|
|
[source,csharp]
|
|
----
|
|
using System;
|
|
using System.Runtime.Serialization;
|
|
|
|
namespace MyLibrary
|
|
{
|
|
public class MyException : Exception
|
|
{
|
|
public MyException()
|
|
{
|
|
}
|
|
|
|
public MyException(string message)
|
|
:base(message)
|
|
{
|
|
}
|
|
|
|
public MyException(string message, Exception innerException)
|
|
: base(message, innerException)
|
|
{
|
|
}
|
|
|
|
protected MyException(SerializationInfo info, StreamingContext context)
|
|
: base(info, context)
|
|
{
|
|
}
|
|
}
|
|
}
|
|
----
|
|
|
|
|
|
ifdef::env-github,rspecator-view[]
|
|
|
|
'''
|
|
== Implementation Specification
|
|
(visible only on this page)
|
|
|
|
=== Message
|
|
|
|
Implement the missing constructors for this exception.
|
|
|
|
|
|
=== Highlighting
|
|
|
|
Exception class declaration
|
|
|
|
|
|
endif::env-github,rspecator-view[]
|