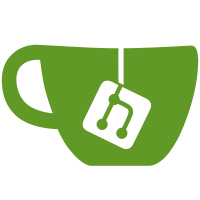
When an include is not surrounded by empty lines, its content is inlined on the same line as the adjacent content. That can lead to broken tags and other display issues. This PR fixes all such includes and introduces a validation step that forbids introducing the same problem again.
72 lines
1.6 KiB
Plaintext
72 lines
1.6 KiB
Plaintext
include::../description.adoc[]
|
|
|
|
include::../ask-yourself.adoc[]
|
|
|
|
include::../recommended.adoc[]
|
|
|
|
== Sensitive Code Example
|
|
|
|
----
|
|
from django.db import models
|
|
from django.db import connection
|
|
from django.db import connections
|
|
from django.db.models.expressions import RawSQL
|
|
|
|
value = input()
|
|
|
|
|
|
class MyUser(models.Model):
|
|
name = models.CharField(max_length=200)
|
|
|
|
|
|
def query_my_user(request, params, value):
|
|
with connection.cursor() as cursor:
|
|
cursor.execute("{0}".format(value)) # Sensitive
|
|
|
|
# https://docs.djangoproject.com/en/2.1/ref/models/expressions/#raw-sql-expressions
|
|
|
|
RawSQL("select col from %s where mycol = %s and othercol = " + value, ("test",)) # Sensitive
|
|
|
|
# https://docs.djangoproject.com/en/2.1/ref/models/querysets/#extra
|
|
|
|
MyUser.objects.extra(
|
|
select={
|
|
'mycol': "select col from sometable here mycol = %s and othercol = " + value}, # Sensitive
|
|
select_params=(someparam,),
|
|
},
|
|
)
|
|
----
|
|
|
|
== Compliant Solution
|
|
|
|
[source,python]
|
|
----
|
|
cursor = connection.cursor(prepared=True)
|
|
sql_insert_query = """ select col from sometable here mycol = %s and othercol = %s """
|
|
|
|
select_tuple = (1, value)
|
|
|
|
cursor.execute(sql_insert_query, select_tuple) # Compliant, the query is parameterized
|
|
connection.commit()
|
|
----
|
|
|
|
include::../see.adoc[]
|
|
|
|
ifdef::env-github,rspecator-view[]
|
|
|
|
'''
|
|
== Implementation Specification
|
|
(visible only on this page)
|
|
|
|
include::../message.adoc[]
|
|
|
|
include::../highlighting.adoc[]
|
|
|
|
'''
|
|
== Comments And Links
|
|
(visible only on this page)
|
|
|
|
include::../comments-and-links.adoc[]
|
|
|
|
endif::env-github,rspecator-view[]
|