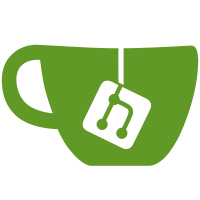
Inline adoc files when they are included exactly once. Also fix language tags because this inlining gives us better information on what language the code is written in.
80 lines
2.1 KiB
Plaintext
80 lines
2.1 KiB
Plaintext
== Why is this an issue?
|
|
|
|
The https://docs.python.org/3/library/stdtypes.html#iterator-types[iterator protocol] specifies that the ``++__iter__++`` method can only return an iterator, i.e. an object with a ``++__next__++`` and an ``++__iter__++`` method (because iterators are also iterable). Returning anything else than an iterator will raise a ``++TypeError++`` as soon as the iteration begins.
|
|
|
|
|
|
Note that https://docs.python.org/3/tutorial/classes.html#generators[generators] and https://docs.python.org/3/tutorial/classes.html#generator-expressions[generator expressions] have both ``++__next__++`` and ``++__iter__++`` methods generated automatically.
|
|
|
|
|
|
This rule raises an issue when the object returned by an ``++__iter__++`` method does not have a method ``++__next__++``.
|
|
|
|
|
|
=== Noncompliant code example
|
|
|
|
[source,python]
|
|
----
|
|
class MyIterable:
|
|
def __init__(self, values):
|
|
self._values = values
|
|
|
|
def __iter__(self):
|
|
return None # Noncompliant
|
|
----
|
|
|
|
|
|
=== Compliant solution
|
|
|
|
[source,python]
|
|
----
|
|
class MyIterable:
|
|
def __init__(self, values):
|
|
self._values = values
|
|
|
|
def __iter__(self):
|
|
return MyIterator(self._values)
|
|
|
|
class MyIterator:
|
|
def __init__(self, values):
|
|
self._values = values
|
|
self._index = 0
|
|
|
|
def __next__(self):
|
|
if self._index >= len(self._values):
|
|
raise StopIteration()
|
|
value = self._values[self._index]
|
|
self._index += 1
|
|
return value
|
|
|
|
def __iter__(self):
|
|
return self
|
|
----
|
|
|
|
|
|
== Resources
|
|
|
|
* https://www.python.org/dev/peps/pep-0234/#python-api-specification[PEP 234 - Iterators]
|
|
* https://docs.python.org/3/library/stdtypes.html#iterator-types[Python documentation - Iterator Types]
|
|
|
|
|
|
ifdef::env-github,rspecator-view[]
|
|
|
|
'''
|
|
== Implementation Specification
|
|
(visible only on this page)
|
|
|
|
=== Message
|
|
|
|
* Return an object complying with iterator protocol.
|
|
|
|
|
|
'''
|
|
== Comments And Links
|
|
(visible only on this page)
|
|
|
|
=== is related to: S5625
|
|
|
|
=== on 28 Apr 2015, 14:21:43 Ann Campbell wrote:
|
|
back to you [~elena.vilchik] for a last check.
|
|
|
|
endif::env-github,rspecator-view[]
|