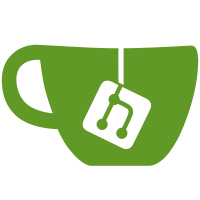
Inline adoc files when they are included exactly once. Also fix language tags because this inlining gives us better information on what language the code is written in.
127 lines
2.7 KiB
Plaintext
127 lines
2.7 KiB
Plaintext
== Why is this an issue?
|
|
|
|
include::../description.adoc[]
|
|
|
|
=== Noncompliant code example
|
|
|
|
There is no way to disable certificate verification in tls, https and request modules but it is possible to not reject request when verification fails.
|
|
|
|
|
|
https://nodejs.org/api/https.html[https] built-in module:
|
|
|
|
[source,javascript]
|
|
----
|
|
let options = {
|
|
hostname: 'www.example.com',
|
|
port: 443,
|
|
path: '/',
|
|
method: 'GET',
|
|
secureProtocol: 'TLSv1_2_method',
|
|
rejectUnauthorized: false ; // Noncompliant
|
|
};
|
|
|
|
let req = https.request(options, (res) => {
|
|
res.on('data', (d) => {
|
|
process.stdout.write(d);
|
|
});
|
|
}); // Noncompliant
|
|
----
|
|
|
|
https://nodejs.org/api/tls.html[tls] built-in module:
|
|
|
|
[source,javascript]
|
|
----
|
|
let options = {
|
|
secureProtocol: 'TLSv1_2_method',
|
|
rejectUnauthorized: false ; // Noncompliant
|
|
};
|
|
|
|
let socket = tls.connect(443, "www.example.com", options, () => {
|
|
process.stdin.pipe(socket);
|
|
process.stdin.resume();
|
|
}); // Noncompliant
|
|
----
|
|
|
|
https://www.npmjs.com/package/request[request] module:
|
|
|
|
[source,javascript]
|
|
----
|
|
let socket = request.get({
|
|
url: 'www.example.com',
|
|
secureProtocol: 'TLSv1_2_method',
|
|
rejectUnauthorized: false ; // Noncompliant
|
|
});
|
|
----
|
|
|
|
=== Compliant solution
|
|
|
|
https://nodejs.org/api/https.html[https] built-in module:
|
|
|
|
[source,javascript]
|
|
----
|
|
let options = {
|
|
hostname: 'www.example.com',
|
|
port: 443,
|
|
path: '/',
|
|
method: 'GET',
|
|
secureProtocol: 'TLSv1_2_method'
|
|
};
|
|
|
|
let req = https.request(options, (res) => {
|
|
res.on('data', (d) => {
|
|
process.stdout.write(d);
|
|
});
|
|
}); // Compliant: by default rejectUnauthorized is set to true
|
|
----
|
|
|
|
|
|
https://nodejs.org/api/tls.html[tls] built-in module:
|
|
|
|
[source,javascript]
|
|
----
|
|
let options = {
|
|
secureProtocol: 'TLSv1_2_method'
|
|
};
|
|
|
|
let socket = tls.connect(443, "www.example.com", options, () => {
|
|
process.stdin.pipe(socket);
|
|
process.stdin.resume();
|
|
}); // Compliant: by default rejectUnauthorized is set to true
|
|
----
|
|
|
|
https://www.npmjs.com/package/request[request] module:
|
|
|
|
[source,javascript]
|
|
----
|
|
let socket = request.get({
|
|
url: 'https://www.example.com/',
|
|
secureProtocol: 'TLSv1_2_method' // Compliant
|
|
}); // Compliant: by default rejectUnauthorized is set to true
|
|
----
|
|
|
|
include::../see.adoc[]
|
|
|
|
ifdef::env-github,rspecator-view[]
|
|
|
|
'''
|
|
== Implementation Specification
|
|
(visible only on this page)
|
|
|
|
include::../message.adoc[]
|
|
|
|
=== Highlighting
|
|
|
|
When ``++request.get/post/...++`` is used:
|
|
|
|
* primary location: the call to ``++request.get/post/...++``
|
|
* secondary location: on ``++rejectUnauthorized: false++``
|
|
** message: 'Set "rejectUnauthorized" to "true".'
|
|
|
|
|
|
'''
|
|
== Comments And Links
|
|
(visible only on this page)
|
|
|
|
include::../comments-and-links.adoc[]
|
|
endif::env-github,rspecator-view[]
|