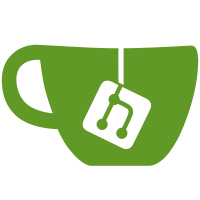
When an include is not surrounded by empty lines, its content is inlined on the same line as the adjacent content. That can lead to broken tags and other display issues. This PR fixes all such includes and introduces a validation step that forbids introducing the same problem again.
94 lines
1.7 KiB
Plaintext
94 lines
1.7 KiB
Plaintext
== Why is this an issue?
|
|
|
|
The purpose of an abstract class is to provide some heritable behaviors while also defining methods which must be implemented by sub-classes.
|
|
|
|
|
|
A ``++class++`` with no abstract methods that was made ``++abstract++`` purely to prevent instantiation should be converted to a concrete ``++class++`` (i.e. remove the ``++abstract++`` keyword) with a ``++protected++`` constructor.
|
|
|
|
|
|
A ``++class++`` with only ``++abstract++`` methods and no inheritable behavior should be converted to an ``++interface++``.
|
|
|
|
=== Noncompliant code example
|
|
|
|
[source,csharp]
|
|
----
|
|
public abstract class Animal //Noncompliant; should be an interface
|
|
{
|
|
abstract void Move();
|
|
abstract void Feed();
|
|
}
|
|
|
|
public abstract class Color //Noncompliant; should be concrete with a protected constructor
|
|
{
|
|
private int red = 0;
|
|
private int green = 0;
|
|
private int blue = 0;
|
|
|
|
public int GetRed()
|
|
{
|
|
return red;
|
|
}
|
|
}
|
|
----
|
|
|
|
=== Compliant solution
|
|
|
|
[source,csharp]
|
|
----
|
|
public interface Animal
|
|
{
|
|
void Move();
|
|
void Feed();
|
|
}
|
|
|
|
public class Color
|
|
{
|
|
private int red = 0;
|
|
private int green = 0;
|
|
private int blue = 0;
|
|
|
|
protected Color()
|
|
{}
|
|
|
|
public int GetRed()
|
|
{
|
|
return red;
|
|
}
|
|
}
|
|
|
|
public abstract class Lamp
|
|
{
|
|
private bool switchLamp = false;
|
|
|
|
public abstract void Glow();
|
|
|
|
public void FlipSwitch()
|
|
{
|
|
switchLamp = !switchLamp;
|
|
if (switchLamp)
|
|
{
|
|
Glow();
|
|
}
|
|
}
|
|
}
|
|
----
|
|
|
|
ifdef::env-github,rspecator-view[]
|
|
|
|
'''
|
|
== Implementation Specification
|
|
(visible only on this page)
|
|
|
|
=== Message
|
|
|
|
Convert this "abstract" (class|record) to (an interface|a concrete type with a private constructor).
|
|
|
|
|
|
'''
|
|
== Comments And Links
|
|
(visible only on this page)
|
|
|
|
include::../comments-and-links.adoc[]
|
|
|
|
endif::env-github,rspecator-view[]
|