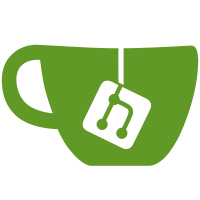
Inline adoc files when they are included exactly once. Also fix language tags because this inlining gives us better information on what language the code is written in.
116 lines
2.0 KiB
Plaintext
116 lines
2.0 KiB
Plaintext
== Why is this an issue?
|
|
|
|
This rule raises an issue when a disposable type contains fields of the following types and does not implement a finalizer:
|
|
|
|
* ``++System.IntPtr++``
|
|
* ``++System.UIntPtr++``
|
|
* ``++System.Runtime.InteropService.HandleRef++``
|
|
|
|
|
|
=== Noncompliant code example
|
|
|
|
[source,csharp]
|
|
----
|
|
using System;
|
|
using System.Runtime.InteropServices;
|
|
|
|
namespace MyLibrary
|
|
{
|
|
public class Foo : IDisposable // Noncompliant: Doesn't have a finalizer
|
|
{
|
|
private IntPtr myResource;
|
|
private bool disposed = false;
|
|
|
|
protected virtual void Dispose(bool disposing)
|
|
{
|
|
if (!disposed)
|
|
{
|
|
// Dispose of resources held by this instance.
|
|
FreeResource(myResource);
|
|
disposed = true;
|
|
|
|
// Suppress finalization of this disposed instance.
|
|
if (disposing)
|
|
{
|
|
GC.SuppressFinalize(this);
|
|
}
|
|
}
|
|
}
|
|
|
|
public void Dispose() {
|
|
Dispose(true);
|
|
}
|
|
}
|
|
}
|
|
----
|
|
|
|
|
|
=== Compliant solution
|
|
|
|
[source,csharp]
|
|
----
|
|
using System;
|
|
using System.Runtime.InteropServices;
|
|
|
|
namespace MyLibrary
|
|
{
|
|
public class Foo : IDisposable
|
|
{
|
|
private IntPtr myResource;
|
|
private bool disposed = false;
|
|
|
|
protected virtual void Dispose(bool disposing)
|
|
{
|
|
if (!disposed)
|
|
{
|
|
// Dispose of resources held by this instance.
|
|
FreeResource(myResource);
|
|
disposed = true;
|
|
|
|
// Suppress finalization of this disposed instance.
|
|
if (disposing)
|
|
{
|
|
GC.SuppressFinalize(this);
|
|
}
|
|
}
|
|
}
|
|
|
|
~Foo()
|
|
{
|
|
Dispose(false);
|
|
}
|
|
}
|
|
}
|
|
----
|
|
|
|
|
|
== Resources
|
|
|
|
* Related: S3881 - "IDisposable" should be implemented correctly
|
|
|
|
|
|
|
|
ifdef::env-github,rspecator-view[]
|
|
|
|
'''
|
|
== Implementation Specification
|
|
(visible only on this page)
|
|
|
|
=== Message
|
|
|
|
Implement a finalizer that calls your "Dispose" method.
|
|
|
|
|
|
=== Highlighting
|
|
|
|
The class declaration
|
|
|
|
|
|
'''
|
|
== Comments And Links
|
|
(visible only on this page)
|
|
|
|
=== is related to: S3881
|
|
|
|
endif::env-github,rspecator-view[]
|